La tokenización asegura que ningún dato sensible de la tarjeta necesite estar en su servidor para que su integración pueda operar de manera compatible con PCI. Si alguna información de la tarjeta pasara por, o se llegase a almacenar en su servidor, usted sería responsable de cualquier penalidad o auditoría que imponga PCI DSS.
DEMO ADD CARD SDK
Github Repository
Revise el ejemplo funcionando
Instalación
Primero necesitas incluir jQuery y los archivos payment_[version].min.js
y payment_[version].min.css
dentro de tu página web especificando "UTF-8" como charset.
<script src="https://code.jquery.com/jquery-1.11.3.min.js" charset="UTF-8"></script>
<link href="https://cdn.globalpay.com.co/ccapi/sdk/payment_stable.min.css" rel="stylesheet" type="text/css" />
<script src="https://cdn.globalpay.com.co/ccapi/sdk/payment_stable.min.js" charset="UTF-8"></script>
Uso
Para revisar ejemplos funcionales utilizando GlobalPayRedebanJS, revisa los ejemplos dentro de este proyecto.
Utilizando el Form de GlobalPay Redeban
Cualquier elemento con la clase payment-form
será automáticamente convertido en una entrada de tarjeta de crédito básica con fecha de vencimiento y el check de CVC.
La manera más fácil de comenzar con la GlobalPayRedebanForm es insertando el siguiente pedazo de código:
<div class="payment-form" id="my-card" data-capture-name="true"></div>
Para obtener el objeto Card
de la instancia PaymentForm
, pregunte al formulario por su tarjeta.
let myCard = $('#my-card');
let cardToSave = myCard.PaymentForm('card');
if(cardToSave == null){
alert("Invalid Card Data");
}
Si la tarjeta (Card
) regresada es null, el estado de error mostrará los campos que necesitan ser arreglados.
Una vez que obtengas un objeto no null de la tarjeta (Card
) del widget, podrás llamar addCard.
Biblioteca Init
Siempre debes inicializar la biblioteca.
/**
* Init library
*
* @param env_mode `prod`, `stg`, `local` para cambiar ambiente. Por defecto es `stg`
* @param client_app_code proporcionado por GlobalPay Redeban.
* @param client_app_key proporcionado por GlobalPay Redeban.
*/
Payment.init('stg', 'CLIENT_APP_CODE', 'CLIENT_APP_KEY');
addCard Agregar una Tarjeta
La función addCard convierte los datos confidenciales de una tarjeta, en un token que puede pasar de forma segura a su servidor, para realizar el cobro al usuario.
Esta funcionalidad consume el servicio de nuestro API pero de manera segura para comercios que no cuentan con certificación PCI.
Aquí podras encontrar la descripción de cada campo en la respuesta.
/*
* @param uid Identificador del usuario. Este es el id que usas del lado de tu aplicativo.
* @param email Email del usuario para iniciar la compra. Usar el formato válido para e-mail.
* @param card La tarjeta que se desea tokenizar.
* @param success_callback Funcionalidad a ejecutar cuando el servicio de la pasarela responde correctamente. (Incluso si se recibe un estado diferente a "valid")
* @param failure_callback Funcionalidad a ejecutar cuando el servicio de la pasarela responde con un error.
* @param payment_form Instancia del formulario de Pago
*/
Payment.addCard(uid, email, cardToSave, successHandler, errorHandler, myCard);
let successHandler = function(cardResponse) {
console.log(cardResponse.card);
if(cardResponse.card.status === 'valid'){
$('#messages').html('Tarjeta correctamente agregada<br>'+
'Estado: ' + cardResponse.card.status + '<br>' +
"Token: " + cardResponse.card.token + "<br>" +
"Referencia de transacción: " + cardResponse.card.transaction_reference
);
}else if(cardResponse.card.status === 'review'){
$('#messages').html('Tarjeta en revisión<br>'+
'Estado: ' + cardResponse.card.status + '<br>' +
"Token: " + cardResponse.card.token + "<br>" +
"Referencia de transacción: " + cardResponse.card.transaction_reference
);
}else{
$('#messages').html('Error<br>'+
'Estado: ' + cardResponse.card.status + '<br>' +
"Mensaje: " + cardResponse.card.message + "<br>"
);
}
submitButton.removeAttr("disabled");
submitButton.text(submitInitialText);
};
let errorHandler = function(err) {
console.log(err.error);
$('#messages').html(err.error.type);
submitButton.removeAttr("disabled");
submitButton.text(submitInitialText);
};
El tercer argumento para el addCard es un objeto Card que contiene los campos requeridos para realizar la tokenización.
getSessionId
El Session ID es un parámetro que GlobalPay Redeban utiliza para fines del antifraude.
Llame este método si usted desea recolectar la información del dispositivo del usuario.
let session_id = Payment.getSessionId();
Una vez que tenga el Session ID, puedes pasarlo a tu servidor para realizar el cargo al usuario.
PaymentForm Referencia Completa
Inserción Manual
Si desea alterar manualmente los campos utilizados por PaymentForm para añadir clases adicionales, el placeholder o id. Puedes rellenar previamente los campos del formulario como se muestra a continuación.
Esto podría ser útil en caso de que desees procesar el formulario en otro idioma (de forma predeterminada, el formulario se representa en español), o para hacer referencia a alguna entrada por nombre o id.
Por ejemplo si desea mostrar el formulario en Inglés y añadir una clase personalizada para el card_number
<div class="payment-form">
<input class="card-number my-custom-class" name="card-number" placeholder="Card number">
<input class="name" id="the-card-name-id" placeholder="Card Holders Name">
<input class="expiry-month" name="expiry-month">
<input class="expiry-year" name="expiry-year">
<input class="cvc" name="cvc">
</div>
Seleccionar Campos
Puedes determinar los campos que mostrará el formulario.
Field |
Description |
data-capture-name |
Input para nombre del Tarjetahabiente, requerido para tokenizar |
data-capture-email |
Input para email del usuario |
data-capture-cellphone |
Input para teléfono celular del usuario |
data-icon-colour |
Color de los íconos |
data-use-dropdowns |
Utiliza una lista desplegable para establecer la fecha de expiración de la tarjeta |
data-exclusive-types |
Define los tipos de tarjetas permitidos |
data-invalid-card-type-message |
Define un mensaje personalizado para mostrar cuando se registre una tarjeta no permitida |
El campo 'data-use-dropdowns' puede resolver el problema que se presenta con la mascara de expiración en dispositivos móviles antiguos.
Se integra en el form de manera simple, como se muestra a continuación:
<div class="payment-form"
id="my-card"
data-capture-name="true"
data-capture-email="true"
data-capture-cellphone="true"
data-icon-colour="#569B29"
data-use-dropdowns="true">
Tipos de tarjetas específicos
Si deseas especificar los tipos de tarjetas permitidos en el formulario, como Exito o Alkosto. Puedes configurarlo como en el siguiente ejemplo:
Cuando una tarjeta de un tipo no permitido es capturado, el formulario se reinicia, bloqueando las entradas y mostrando un mensaje
Tipo de tarjeta invalida para está operación.
<div class="payment-form"
id="my-card"
data-capture-name="true"
data-exclusive-types="ex,ak"
data-invalid-card-type-message="Tarjeta invalida. Por favor ingresa una tarjeta Exito / Alkosto."
>
Revisa todos los tipos de tarjetas permitidos por GlobalPay Redeban.
Leyendo los Valores
PaymentForm proporciona funcionalidad que le permite leer los valores del campo de formulario directamente con JavaScript.
Genera un elemento de PaymentForm y asigne un id único (en este ejemplo my-card
)
<div class="payment-form" id="my-card" data-capture-name="true"></div>
El siguiente javascript muestra cómo leer cada valor del formulario en variables locales.
let myCard = $('#my-card');
let cardType = myCard.PaymentForm('cardType');
let name = myCard.PaymentForm('name');
let expiryMonth = myCard.PaymentForm('expiryMonth');
let expiryYear = myCard.PaymentForm('expiryYear');
let fiscalNumber = myCard.PaymentForm('fiscalNumber');
Funciones
Para llamar a una función en un elemento PaymentForm, sigue el patrón a continuación.
Remplace el texto 'function' con el nombre de la función que desea llamar.
$('#my-card').PaymentForm('function')
Las funciones disponibles se enumeran a continuación
Function |
Description |
card |
Obtiene la tarjeta del objeto |
cardType |
Obtiene el tipo de tarjeta que se capturó |
name |
Obtiene el nombre capturado |
expiryMonth |
Obtiene el mes de expiración de la tarjeta |
expiryYear |
Obtiene el año de expiración de la tarjeta |
fiscalNumber |
Obtiene el número fiscal del usuario / cédula |
Función CardType
La función cardType
devolverá una cadena según el número de tarjeta ingresado. Si no se puede determinar el tipo de tarjeta, se le dará una cadena vacía.
Marcas permitidas
Github Repository
Redeban Payment Android SDK is a library that allows developers to easily connect to the Redeban CREDITCARDS API
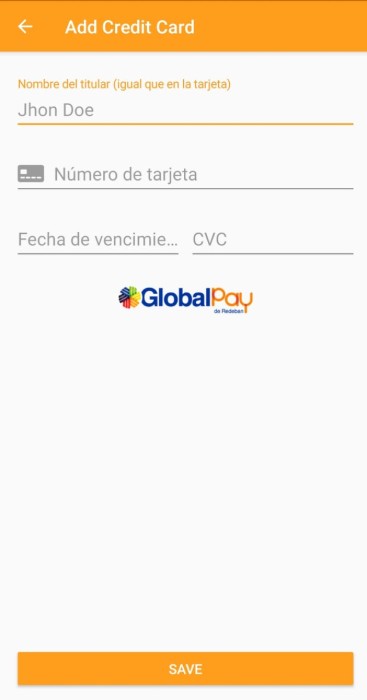
Installation
Android Studio (or Gradle)
Add it in your root build.gradle at the end of repositories:
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Add this line to your app's build.gradle
inside the dependencies
section:
implementation 'com.github.globalpayredeban:globalpayredeban-android:1.2.9'
ProGuard
If you're planning on optimizing your app with ProGuard, make sure that you exclude the Redeban bindings. You can do this by adding the following to your app's proguard.cfg
file:
-keep class com.redeban.payment.** { *; }
Usage
Using the CardMultilineWidget
You can add a widget to your apps that easily handles the UI states for collecting card data.
First, add the CardMultilineWidget to your layout.
<com.redeban.payment.view.CardMultilineWidget
android:id="@+id/card_multiline_widget"
android:layout_alignParentTop="true"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
You can customize the view with this tags:
app:shouldShowPostalCode="true"
app:shouldShowRedebanLogo="true"
app:shouldShowCardHolderName="true"
app:shouldShowScanCard="true"
In order to use any of this tags, you'll need to enable the app XML namespace somewhere in the layout.
xmlns:app="http://schemas.android.com/apk/res-auto"
To get a Card
object from the CardMultilineWidget
, you ask the widget for its card.
Card cardToSave = cardWidget.getCard();
if (cardToSave == null) {
Alert.show(mContext,
"Error",
"Invalid Card Data");
return;
}
If the returned Card
is null, error states will show on the fields that need to be fixed.
Once you have a non-null Card
object from the widget, you can call addCard.
Init library
You should initialize the library on your Application or in your first Activity.
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import com.redeban.payment.Payment;
import com.redeban.payment.example.utils.Constants;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
/**
* Init library
*
* @param test_mode false to use production environment
* @param redeban_client_app_code provided by Redeban.
* @param redeban_client_app_key provided by Redeban.
*/
Payment.setEnvironment(Constants.REDEBAN_IS_TEST_MODE, Constants.REDEBAN_CLIENT_APP_CODE, Constants.REDEBAN_CLIENT_APP_KEY);
// In case you have your own Fraud Risk Merchant Id
//Payment.setRiskMerchantId(1000);
// Note: for most of the devs, that's not necessary.
}
}
addCard
addCard converts sensitive card data to a single-use token which you can safely pass to your server to charge the user.
Payment.addCard(mContext, uid, email, cardToSave, new TokenCallback() {
public void onSuccess(Card card) {
if(card != null){
if(card.getStatus().equals("valid")){
Alert.show(mContext,
"Card Successfully Added",
"status: " + card.getStatus() + "\n" +
"Card Token: " + card.getToken() + "\n" +
"transaction_reference: " + card.getTransactionReference());
} else if (card.getStatus().equals("review")) {
Alert.show(mContext,
"Card Under Review",
"status: " + card.getStatus() + "\n" +
"Card Token: " + card.getToken() + "\n" +
"transaction_reference: " + card.getTransactionReference());
} else {
Alert.show(mContext,
"Error",
"status: " + card.getStatus() + "\n" +
"message: " + card.getMessage());
}
}
//TODO: Create charge or Save Token to your backend
}
public void onError(RedebanError error) {
Alert.show(mContext,
"Error",
"Type: " + error.getType() + "\n" +
"Help: " + error.getHelp() + "\n" +
"Description: " + error.getDescription());
//TODO: Handle error
}
});
The first argument to addCard is mContext (Context).
+ mContext. Context of the Current Activity
The second argument to addCard is uid (String).
+ uid Customer identifier. This is the identifier you use inside your application; you will receive it in notifications.
The third argument to addCard is email (String).
+ email Email of the customer
The fourth argument to addCard is a Card object. A Card contains the following fields:
- number: card number as a string without any separators, e.g. '4242424242424242'.
- holderName: cardholder name.
- expMonth: integer representing the card's expiration month, e.g. 12.
- expYear: integer representing the card's expiration year, e.g. 2013.
- cvc: card security code as a string, e.g. '123'.
- type:
The fifth argument tokenCallback is a callback you provide to handle responses from Redeban.
It should send the token to your server for processing onSuccess, and notify the user onError.
Here's a sample implementation of the token callback:
Payment.addCard(
mContext, uid, email, cardToSave,
new TokenCallback() {
public void onSuccess(Card card) {
// Send token to your own web service
MyServer.chargeToken(card.getToken());
}
public void onError(RedebanError error) {
Toast.makeText(getContext(),
error.getDescription(),
Toast.LENGTH_LONG).show();
}
}
);
addCard
is an asynchronous call – it returns immediately and invokes the callback on the UI thread when it receives a response from Redeban's servers.
getSessionId
The Session ID is a parameter Redeban use for fraud purposes.
Call this method if you want to Collect your user's Device Information.
String session_id = Payment.getSessionId(mContext);
Once you have the Session ID, you can pass it to your server to charge the user.
Client-side validation helpers
The Card object allows you to validate user input before you send the information to Redeban.
validateNumber
Checks that the number is formatted correctly and passes the Luhn check.
validateExpiryDate
Checks whether or not the expiration date represents an actual month in the future.
validateCVC
Checks whether or not the supplied number could be a valid verification code.
validateCard
Convenience method to validate card number, expiry date and CVC.
Getting started with the Android example app
Note: the app require an Android SDK and Gradle to build and run.
Building and Running the RedebanStore
Before you can run the RedebanStore application, you need to provide it with your Redeban Credentials and a Sample Backend.
- If you don't have any Credentials yet, please ask your contact on Redeban Team for it.
- Head to https://github.com/redeban/example-java-backend and click "Deploy to Heroku" (you may have to sign up for a Heroku account as part of this process). Provide your Redeban Server Credentials
REDEBAN_SERVER_APP_CODE
and REDEBAN_SERVER_APP_KEY
fields under 'Env'. Click "Deploy for Free".
- Open the project on Android Studio.
- Replace the
REDEBAN_CLIENT_APP_CODE
and REDEBAN_CLIENT_APP_KEY
constants in Constants.java with your own Redeban Client Credentials.
- Replace the
BACKEND_URL
variable in the Constants.java file with the app URL Heroku provides you with (e.g. "https://my-example-app.herokuapp.com")
- Run the Project.
Important Note: if you only have one APP_CODE, please asume that it's your REDEBAN_SERVER_APP_CODE
. So you need to ask your contact on Redeban Team for your REDEBAN_CLIENT_APP_CODE
.
===================
El SDK Android de pagos Redeban es una biblioteca que permite a los desarrolladores conectarse fácilmente al API de tarjetas de Crédito de Redeban.
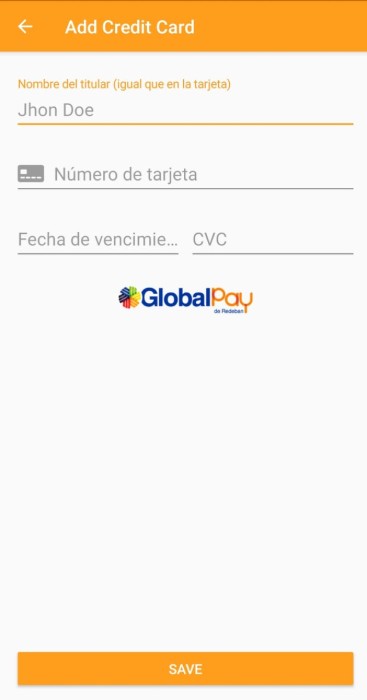
Instalación
Android Studio (o Gradle)
Agrege esta línea en su aplicación build.gradle
dentro de la sección dependencies
:
implementation 'com.redeban:payment-android:1.2.8'
ProGuard
Si usted está planeando optimiar su aplicación con ProGuard, asegúrese de excluir los enlaces de Redeban. Ustede puede realizarlo añadiendo lo siguiente al archivo proguard.cfg
de su app:
-keep class com.redeban.payment.** { *; }
Uso
Utilizando el CardMultilineWidget
Puede agregar un widget a sus aplicaciones que maneje fácilmente los estados de la interfaz de usuario para recopilar datos de la tarjeta.
Primero, añada el CardMultilineWidget a su layout.
<com.redeban.payment.view.CardMultilineWidget
android:id="@+id/card_multiline_widget"
android:layout_alignParentTop="true"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
Ustede puede personalizar la vista con las siguientes etiquetas:
app:shouldShowPostalCode="true"
app:shouldShowPaymentezLogo="true"
app:shouldShowCardHolderName="true"
app:shouldShowScanCard="true"
Para usar cualquiera de estas etiquetas, deberá habilitar el espacio de nombres XML de la aplicación en algún lugar del layout.
xmlns:app="http://schemas.android.com/apk/res-auto"
Para obtener un objeto Card
delCardMultilineWidget
, pidale al widget su tarjeta.
Card cardToSave = cardWidget.getCard();
if (cardToSave == null) {
Alert.show(mContext,
"Error",
"Invalid Card Data");
return;
}
Si la Card
devuelta es null , se mostrarán estados de error en los campos que deben corregirse.
Una vez que tenga un objeto Card
no null regresado desde el widget, puede llamar a addCard.
Inicializar Biblioteca
Usted debe inicializar la biblioteca en su Aplicación en su primera actividad.
You should initialize the library on your Application or in your first Activity.
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import com.redeban.payment.Payment;
import com.redeban.payment.example.utils.Constants;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
/**
* Init library
*
* @param test_mode false to use production environment
* @param redeban_client_app_code provided by Redeban.
* @param redeban_client_app_key provided by Redeban.
*/
Payment.setEnvironment(Constants.REDEBAN_IS_TEST_MODE, Constants.REDEBAN_CLIENT_APP_CODE, Constants.REDEBAN_CLIENT_APP_KEY);
// In case you have your own Fraud Risk Merchant Id
//Payment.setRiskMerchantId(1000);
// Note: for most of the devs, that's not necessary.
}
}
addCard
addCard convierte datos confidenciales de la tarjeta en un token de un solo uso que puede pasar de forma segura a su servidor para realizar el cobro al usuario.
Payment.addCard(mContext, uid, email, cardToSave, new TokenCallback() {
public void onSuccess(Card card) {
if(card != null){
if(card.getStatus().equals("valid")){
Alert.show(mContext,
"Card Successfully Added",
"status: " + card.getStatus() + "\n" +
"Card Token: " + card.getToken() + "\n" +
"transaction_reference: " + card.getTransactionReference());
} else if (card.getStatus().equals("review")) {
Alert.show(mContext,
"Card Under Review",
"status: " + card.getStatus() + "\n" +
"Card Token: " + card.getToken() + "\n" +
"transaction_reference: " + card.getTransactionReference());
} else {
Alert.show(mContext,
"Error",
"status: " + card.getStatus() + "\n" +
"message: " + card.getMessage());
}
}
//TODO: Create charge or Save Token to your backend
}
public void onError(RedebanError error) {
Alert.show(mContext,
"Error",
"Type: " + error.getType() + "\n" +
"Help: " + error.getHelp() + "\n" +
"Description: " + error.getDescription());
//TODO: Handle error
}
});
El primer argumento del addCard es mContext (Context).
+ mContext. Context de la Actividad actual.
El segundo argumento del addCard es uid (Cadena).
+ uid Identificador de comprador. Este es el identificador que usa dentro de su aplicación; lo recibirá en las notificaciones.
El tercer argumento del addCard es el email (Cadena).
+ email Email del comprador
El cuarto argumento del addCard es un objeto de tipo Card. Un objeto Card contiene los siguientes campos:
- number: número de tarjeta como cadena sin ningún separador, por ejemplo '4242424242424242'.
- holderName: nombre del tarjehabiente.
- expMonth: entero que representa el mes de expiración de la tarjeta, por ejemplo 12.
- expYear: entero que represetna el año de expiración de la tarjeta, por ejemplo 2019.
- cvc: código de seguridad de la tarjeta, como cadena, por ejemplo '123'.
- type: el tipo de tarjeta.
El quinto argumento tokenCallBack es un callback que usted provee para manejar las respuestas recibidas de Redeban.
The fifth argument tokenCallback is a callback you provide to handle responses from Redeban.
Deberá enviar el token a su servidor para procesar onSuccess y notificar al usuario onError.
Aquí se muestra un ejemplo de implementación de callback del token:
Payment.addCard(
mContext, uid, email, cardToSave,
new TokenCallback() {
public void onSuccess(Card card) {
// Send token to your own web service
MyServer.chargeToken(card.getToken());
}
public void onError(RedebanError error) {
Toast.makeText(getContext(),
error.getDescription(),
Toast.LENGTH_LONG).show();
}
}
);
addCard
es una llamada asíncrona - regresa inmediatamente e invoca el callback en el hilo de la interfaz, cuando recibe una respuesta de los servidores de Redeban.
getSessionId
El Session ID es un parámetro que Redeban utiliza para fines de antifraude.
Llave esté método cuando quiera recabar la información del dispositivo.
String session_id = Payment.getSessionId(mContext);
Una vez que tenga el Session ID, usted podrá pasarlo a su servidor para realizar el cobro a su usuario.
Herramientas de validación del lado del Cliente
El objeto Card permite validar la información capturada por el usuario antes de enviarla a Redeban.
validateNumber
Verifica que el número tenga el formato correcto y pase el algoritmo de Luhn.
validateExpiryDate
Comprueba que la fecha de expiración representa una fecha real futura.
validateCVC
Comprueba si el número proporcionado podría ser un código de verificación válido o no.
validateCard
Método que valida el número de tarjeta, la fecha de expiración y el CVC.
Introducción a la aplicación de ejemplo de Android
Nota: la aplicación requiere Android SDK y Gradle para coinstruir y ejecutar.
Construyendo y Ejecutando la PaymentStore
Antes de que pueda pueda correr la aplicación PaymentStore, ested necesita proveerla con las credenciales de Redeban y un backend de muestra.
- Si aún no cuenta con credenciales, pídalas a su contancto en el equipo de Redeban.
- Dirígase a https://github.com/globalpayredeban/example-java-backend y haga click en "Deploy to Heroku" (es posible que tengas que registrarte para obtener una cuenta de Heroku como parte de este proceso). Proporcione sus credenciales de servidor de Redeban
REDEBAN_SERVER_APP_CODE
y REDEBAN_SERVER_APP_KEY
en los campos "Env". Haga click en "Deploy for Free".
- Abra el proyecto en Android Studio.
- Reemplace las constantes
REDEBAN_CLIENT_APP_CODE
y REDEBAN_CLIENT_APP_KEY
en Constants.java con sus propias credenciales de Redeban.
- Reemplace la variable
BACKEND_URL
en el archivo Constants.java con la URL de la aplicación que Heroku le proporciona (por ejemplo," https://my-example-app.herokuapp.com ")
- Ejecute el proyecto.
Nota importante: si solo tiene un APP_CODE, suponga que es su REDEBAN_SERVER_APP_CODE
. Por lo tanto, debe solicitar a su contacto en el equipo de Redeban su REDEBAN_CLIENT_APP_CODE
.
Github Repository
Requirements
Version <= 1.4.x
- iOS 9.0 or Later
- Xcode 9
Version >= 1.5.x
- iOS 9.0 or Later
- Xcode 10
Framework Dependencies:
Accelerate
AudioToolbox
AVFoundation
CoreGraphics
CoreMedia
CoreVideo
Foundation
MobileCoreServices
OpenGLES
QuartzCore
Security
UIKit
CommonCrypto (Just for version 1.4)
Project Configuration
- ObjC in other linker flags in target
- lc++ in target other linker flags
- Disable Bitcode
INSTALLATION
Carthage
If you haven't already, install the latest version of Carthage
Add this to the Cartfile:
git "https://github.com/globalpayredeban/globalpayredeban-ios"
For Beta Versions:
git "https://github.com/globalpayredeban/globalpayredeban-ios" "master"
ObjC configuration
Set ALWAYS_EMBED_SWIFT_STANDARD_LIBRARIES = YES
In Build Phases -> Embed Frameworks Uncheck "Copy Only When Installing"
Manual Installation(Recommended)
SDK is a dynamic framework (More Info) so you have to build each version for the desire target (simulator and device). To make the integration easy, you can follow these instructions in order to have just one Universal .framework file.
- Build the SDK and create .framework files
This will create a /build folder where there are all the necesary .framework (simulator, iphoneos and universal)
- With Target PaymentSDK selected, build the project for any iOS Simulator.
- With Target PaymentSDK selected, build the project for a physical device.
After
- With the Target PaymentezSDK-Universal, build the project for any iOS device.
- Inside the group Products -> PaymentSDK.framework -> Show in finder
- Inside the the directory of PaymentSDK.framework, CMD+up
You can see three groups, inside the group Release-iosuniversal, you'll find the PaymentSDK.framework
Or if you prefer you can download pre-compilled .framework files from Releases
- Drag the PaymentSDK.framework To your project and check "Copy Files if needed".
In Target->General : Add PaymentSDK.framework to Frameworks, Libraries, and Embedded Content
In Target->Build Settings : Validate Workspace should be YES
- Update the Build Settings with
Set ALWAYS_EMBED_SWIFT_STANDARD_LIBRARIES = YES
In Build Phases -> Embed Frameworks Uncheck "Copy Only When Installing"
- If you use the Universal version and you want to upload to the appstore. Add Run Script Phase: Target->Build Phases -> + ->New Run Script Phase. And paste the following. Make sure that this build phase is added after Embed Frameworks phase.
bash "${BUILT_PRODUCTS_DIR}/${FRAMEWORKS_FOLDER_PATH}/PaymentSDK.framework/install_dynamic.sh"
Usage
Importing Swift
import PaymentSDK
Setting up your app inside AppDelegate->didFinishLaunchingWithOptions. You should use the Payment Client Credentials (Just ADD enabled)
PaymentSDKClient.setEnvironment("AbiColApp", secretKey: "2PmoFfjZJzjKTnuSYCFySMfHlOIBz7", testMode: true)
Types of implementation
There are 3 ways to present the Add Card Form:
- As a Widget in a Custom View
- As a Viewcontroller Pushed to your UINavigationController
- As a ViewController presented in Modal
The AddCard Form includes: Card io scan, and card validation.
Show AddCard Widget
In order to create a widget you should create a PaymentAddNativeController from the PaymentSDKClient. Then add it to the UIView that will be the container of the Payment Form. The min height should be 300 px, and whole screen as width (270px without payment logo)
Note: When you are using the Payment Form as Widget. The Client custom ViewController will be responsible for the layout and synchronization (aka Spinner or loading)
The widget can scan with your phones camera the credit card data using card.io.
let paymentAddVC = self.addPaymentWidget(toView: self.addView, delegate: nil, uid:UserModel.uid, email:UserModel.email)
Objc
[self addPaymentWidgetToView:self. addView delegate:self uid:@"myuid" email:@"myemail"];
Retrive the valid credit card from the PaymentAddNativeController (Widget):
if let validCard = paymentAddVC.getValidCard() // CHECK IF THE CARD IS VALID, IF THERE IS A VALIDATION ERROR NIL VALUE WILL BE RETURNED
{
sender?.isEnabled = false
PaymentSDKClient.createToken(validCard, uid: UserModel.uid, email: UserModel.email, callback: { (error, cardAdded) in
if cardAdded != nil // handle the card status
{
}
else if error != nil //handle the error
{
}
})
}
Objc
PaymentCard *validCard = [self.paymentAddVC getValidCard];
if (validCard != nil) // Check if it is avalid card
{
[PaymentSDKClient add:validCard uid:USERMODEL_UID email:USERMODEL_EMAIL callback:^(PaymentSDKError *error, PaymentCard *cardAdded) {
[sender setEnabled:YES];
if(cardAdded != nil) // handle the card status
{
}
else //handle the error
{
}
}];
}
Pushed to your NavigationController
self.navigationController?.pushPaymentViewController(delegate: self, uid: UserModel.uid, email: UserModel.email)
Objc
[self.navigationController pushPaymentViewControllerWithDelegate:self uid:@"myuid" email:@"mymail@mail.com"]`;
Present as Modal
self.presentPaymentViewController(delegate: self, uid: "myuid", email: "myemail@email.com")
Objc
[self presentPaymentViewControllerWithDelegate:self uid:@"myuid" email:@"myemail@email.com"];
PaymentCardAddedDelegate Protocol
If you present the Form as a viewcontroller (push and modal) you must implement the PaymetnezCardAddedDelegate Protocol in order to handle the states or actions inside the Viewcontroller. If you are using Widget implementation you can handle the actions as described above.
protocol PaymentCardAddedDelegate
{
func cardAdded(_ error:PaymentSDKError?, _ cardAdded:PaymentCard?)
func viewClosed()
}
func cardAdded(_ error:PaymentSDKError?, _ cardAdded:PaymentCard?)
is called whenever there is an error or a card is added.
func viewClosed()
Whenever the modal is closed
Scan Card
If you want to do the scan yourself, using card.io
PaymentSDKClient.scanCard(self) { (closed, number, expiry, cvv, card) in
if !closed // user did not closed the scan card dialog
{
if card != nil // paymentcard object to handle the data
{
}
})
-ObjC
[PaymentSDKClient scanCard:self callback:^(BOOL userClosed, NSString *cardNumber, NSString *expiryDate, NSString *cvv, PaymentCard *card) {
if (!userClosed) //user did not close the scan card dialog
{
if (card != nil) // Handle card
{
}
}
}];
Add Card (Only PCI Integrations)
For custom form integrations
Fields required
+ cardNumber: card number as a string without any separators, e.g. 4111111111111111.
+ cardHolder: cardholder name.
+ expuryMonth: integer representing the card's expiration month, 01-12.
+ expiryYear: integer representing the card's expiration year, e.g. 2020.
+ cvc: card security code as a string, e.g. '123'.
let card = PaymentCard.createCard(cardHolder:"Gustavo Sotelo", cardNumber:"4111111111111111", expiryMonth:10, expiryYear:2020, cvc:"123")
if card != nil // A valid card was created
{
PaymentSDKClient.add(card, uid: "69123", email: "gsotelo@globalpay.com", callback: { (error, cardAdded) in
if cardAdded != nil
{
//the request was succesfully sent, you should check the cardAdded status
}
})
}
else
{
//handle invalid card
}
ObjC
PaymentCard *validCard = [PaymentCard createCardWithCardHolder:@"Gustavo Sotelo" cardNumber:@"4111111111111111" expiryMonth:10 expiryYear:2020 cvc:@"123"];
if (validCard != nil) // Check if it is avalid card
{
[PaymentSDKClient add:validCard uid:USERMODEL_UID email:USERMODEL_EMAIL callback:^(PaymentSDKError *error, PaymentCard *cardAdded) {
[sender setEnabled:YES];
if(cardAdded != nil) // handle the card status
{
}
else //handle the error
{
}
}];
}
Secure Session Id
Debit actions should be implemented in your own backend. For security reasons we provide a secure session id generation, for kount fraud systems. This will collect the device information in background
let sessionId = PaymentSDKClient.getSecureSessionId()
Objc
NSString *sessionId = [PaymentSDKClient getSecureSessionId];
Utils
Get Card Assets
let card = PaymentCard.createCard(cardHolder:"Gustavo Sotelo", cardNumber:"4111111111111111", expiryMonth:10, expiryYear:2020, cvc:"123")
if card != nil // A valid card was created
{
let image = card.getCardTypeAsset()
}
Get Card Type (Just Amex, Mastercard, Visa, Diners)
let card = PaymentCard.createCard(cardHolder:"Gustavo Sotelo", cardNumber:"4111111111111111", expiryMonth:10, expiryYear:2020, cvc:"123")
if card != nil // A valid card was created
{
switch(card.cardType)
{
case .amex:
case .masterCard:
case .visa:
case .diners:
default:
//not supported action
}
}
Customize Look & Feel
You can customize widget colors sample
paymentAddVC.baseFontColor = .white
paymentAddVC.baseColor = .green
paymentAddVC.backgroundColor = .white
paymentAddVC.showLogo = false
paymentAddVC.baseFont = UIFont(name: "Your Font", size: 12) ?? UIFont.systemFont(ofSize: 12)
The customizable elements of the form are the following:
baseFontColor
: The color of the font of the fields
baseColor
: Color of the lines and titles of the fields
backgroundColor
: Background color of the widget
showLogo
: Enable or disable Payment Logo
baseFont
: Font of the entire form
nameTitle
: String for the custom placeholder for the Name Field
cardTitle
: String for the custom placeholder for the Card Field
invalidCardTitle
String for the error message when a card number is invalid
Building and Running the PaymentSwift
Before you can run the PaymentStore application, you need to provide it with your APP_CODE, APP_SECRET_KEY and a sample backend.
- If you haven't already and APP_CODE and APP_SECRET_KEY, please ask your contact on Payment Team for it.
- Replace the
PAYMENT_APP_CODE
and PAYMENT_APP_SECRET_KEY
in your AppDelegate as shown in Usage section
- Head to https://github.com/globalpayredeban/example-java-backend and click "Deploy to Heroku" (you may have to sign up for a Heroku account as part of this process). Provide your Payment Server Credentials APP_CODE and APP_SECRET_KEY fields under 'Env'. Click "Deploy for Free".
- Replace the
BACKEND_URL
variable in the MyBackendLib.swift (inside the variable myBackendUrl) with the app URL Heroku provides you with (e.g. "https://my-example-app.herokuapp.com")
- Replace the variables (uid and email) in UserModel.swift with your own user id reference